Export Quads #
Problems #
How can I export my database to files, using standard representational format?
Solution #
This code creates a set of quads, then exports them into several formats:
See the full list of source code
package main
import (
"fmt"
"github.com/cayleygraph/quad"
"reflect"
// Add some predefined vocabularies
"github.com/cayleygraph/quad/voc/rdf"
"github.com/tombenke/cayley-cookbook-src/kbase/voc/foaf"
)
func main() {
// Create Quads about some people using Blank Nodes as references
quadsWithBNodes := makeQuadsWithBNodes()
// Take a look at the resulted quads
printQuads(quadsWithBNodes)
// Create Quads about some people using IRIs as references
quadsWithIRIs := makeQuadsWithIRIs()
// Take a look at the resulted quads
printQuads(quadsWithIRIs)
}
func makeQuadsWithBNodes() []quad.Quad {
label := "people"
quads := []quad.Quad{}
// Generate a Blank Node to represent the person internally
// You can create a sequence of internal IDs for the Blank Nodes
var seq quad.Sequence
luke := seq.Next()
// Alternatively create a Blank Node with a random internal ID
// luke := quad.RandomBlankNode()
quads = append(quads, quad.Make(luke, quad.IRI(rdf.Type), quad.IRI(foaf.Person), label))
quads = append(quads, quad.Make(luke, quad.IRI(foaf.FamilyName), "Luke", label))
quads = append(quads, quad.Make(luke, quad.IRI(foaf.GivenName), "Skywalker", label))
quads = append(quads, quad.Make(luke, quad.IRI(foaf.Age), 23, label))
leia := seq.Next()
quads = append(quads, quad.Make(leia, quad.IRI(rdf.Type), quad.IRI(foaf.Person), label))
quads = append(quads, quad.Make(leia, quad.IRI(foaf.FamilyName), "Leia", label))
quads = append(quads, quad.Make(leia, quad.IRI(foaf.GivenName), "Organa", label))
quads = append(quads, quad.Make(leia, quad.IRI(foaf.Knows), luke, label))
return quads
}
func makeQuadsWithIRIs() []quad.Quad {
label := "people"
quads := []quad.Quad{}
// Create IRIs to represent the person globally, and universally
luke := quad.IRI("https://swapi.co/resource/human/luke_skywalker")
// Alternatively create a Blank Node with a random internal ID
// luke := quad.RandomBlankNode()
quads = append(quads, quad.Make(luke, quad.IRI(rdf.Type), quad.IRI(foaf.Person), label))
quads = append(quads, quad.Make(luke, quad.IRI(foaf.FamilyName), "Luke", label))
quads = append(quads, quad.Make(luke, quad.IRI(foaf.GivenName), "Skywalker", label))
leia := quad.IRI("https://swapi.co/resource/human/leia_organa")
quads = append(quads, quad.Make(leia, quad.IRI(rdf.Type), quad.IRI(foaf.Person), label))
quads = append(quads, quad.Make(leia, quad.IRI(foaf.FamilyName), "Leia", label))
quads = append(quads, quad.Make(leia, quad.IRI(foaf.GivenName), "Organa", label))
quads = append(quads, quad.Make(leia, quad.IRI(foaf.Knows), luke, label))
return quads
}
func printQuads(quads []quad.Quad) {
fmt.Println("The details of the quads created:")
for i, q := range quads {
fmt.Printf("quads[%d]:\n", i)
fmt.Printf(" subject: %s %v\n", q.Get(quad.Subject), reflect.TypeOf(q.Get(quad.Subject)))
fmt.Printf(" predicate: %s %v\n", q.Get(quad.Predicate), reflect.TypeOf(q.Get(quad.Predicate)))
fmt.Printf(" object: %s %v\n", q.Get(quad.Object), reflect.TypeOf(q.Get(quad.Object)))
fmt.Printf(" label: %s %v\n\n", q.Get(quad.Label), reflect.TypeOf(q.Get(quad.Label)))
}
fmt.Println("The quads in NQuad representation:")
for _, q := range quads {
fmt.Printf("%s\n", q.NQuad())
}
}
cd quad/writer
go run export_nquads_to_stdout.go data
The results:
digraph cayley_graph {
"_:n7510155278594416037" -> "<foaf:Person>" [ label = "<rdf:type>" ];
"_:n7510155278594416037" -> "\"Luke\"" [ label = "<foaf:givenName>" ];
"_:n7510155278594416037" -> "\"Skywalker\"" [ label = "<foaf:familyName>" ];
"_:n7510155278594416037" -> "\"23\"^^<xsd:integer>" [ label = "<foaf:age>" ];
"_:n7235299428451853102" -> "<foaf:Person>" [ label = "<rdf:type>" ];
"_:n7235299428451853102" -> "_:n7510155278594416037" [ label = "<foaf:knows>" ];
"_:n7235299428451853102" -> "\"Leia\"" [ label = "<foaf:givenName>" ];
"_:n7235299428451853102" -> "\"Organa\"" [ label = "<foaf:familyName>" ];
}
Creator "Cayley"
graph [ directed 1
node [ id 0 label "_:n7510155278594416037" ]
node [ id 1 label "<foaf:Person>" ]
edge [ source 0 target 1 label "<rdf:type>" ]
node [ id 2 label ""Luke"" ]
edge [ source 0 target 2 label "<foaf:givenName>" ]
node [ id 3 label ""Skywalker"" ]
edge [ source 0 target 3 label "<foaf:familyName>" ]
node [ id 4 label ""23"^^<xsd:integer>" ]
edge [ source 0 target 4 label "<foaf:age>" ]
node [ id 5 label "_:n7235299428451853102" ]
edge [ source 5 target 1 label "<rdf:type>" ]
edge [ source 5 target 0 label "<foaf:knows>" ]
node [ id 6 label ""Leia"" ]
edge [ source 5 target 6 label "<foaf:givenName>" ]
node [ id 7 label ""Organa"" ]
edge [ source 5 target 7 label "<foaf:familyName>" ]
]
<?xml version="1.0" encoding="UTF-8"?>
<graphml xmlns="http://graphml.graphdrawing.org/xmlns"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://graphml.graphdrawing.org/xmlns
http://www.yworks.com/xml/schema/graphml/1.1/ygraphml.xsd"
xmlns:y="http://www.yworks.com/xml/graphml">
<key id="d0" for="node" yfiles.type="nodegraphics"/>
<key id="d1" for="edge" yfiles.type="edgegraphics"/>
<graph id="G" edgedefault="directed">
<node id="n0"><data key="d0">
<y:ShapeNode>
<y:Geometry x="170.5" y="-15.0" width="220.0" height="30.0"/>
<y:Fill color="#E1E1E1" transparent="false"/>
<y:BorderStyle type="line" width="1.0" color="#000000"/>
<y:NodeLabel>_:n7510155278594416037</y:NodeLabel>
<y:Shape type="ellipse"/>
</y:ShapeNode>
</data></node>
<node id="n1"><data key="d0">
<y:ShapeNode>
<y:Geometry x="170.5" y="-15.0" width="130.0" height="30.0"/>
<y:Fill color="#FFFF99" transparent="false"/>
<y:BorderStyle type="line" width="1.0" color="#000000"/>
<y:NodeLabel><foaf:Person></y:NodeLabel>
<y:Shape type="ellipse"/>
</y:ShapeNode>
</data></node>
<edge source="n0" target="n1"><data key="d1">
<y:PolyLineEdge>
<y:Path sx="0.0" sy="-15.0" tx="29.5" ty="0.0">
<y:Point x="425.0" y="0.0"/>
</y:Path>
<y:LineStyle type="line" width="1.0" color="#000000"/>
<y:Arrows source="none" target="standard"/>
<y:EdgeLabel alignment="center" backgroundColor="#FFFFFF" configuration="AutoFlippingLabel" distance="2.0" fontFamily="Dialog" fontSize="12" fontStyle="plain" hasLineColor="false" height="17.96875" horizontalTextPosition="center" iconTextGap="4" modelName="centered" preferredPlacement="anywhere" ratio="0.5" textColor="#000000" verticalTextPosition="bottom" visible="true"><rdf:type></y:EdgeLabel>
<y:BendStyle smoothed="false"/>
</y:PolyLineEdge>
</data></edge>
<node id="n2"><data key="d0">
<y:ShapeNode>
<y:Geometry x="170.5" y="-15.0" width="60.0" height="30.0"/>
<y:Fill color="#CCFFCC" transparent="false"/>
<y:BorderStyle type="line" width="1.0" color="#000000"/>
<y:NodeLabel>"Luke"</y:NodeLabel>
<y:Shape type="ellipse"/>
</y:ShapeNode>
</data></node>
<edge source="n0" target="n2"><data key="d1">
<y:PolyLineEdge>
<y:Path sx="0.0" sy="-15.0" tx="29.5" ty="0.0">
<y:Point x="425.0" y="0.0"/>
</y:Path>
<y:LineStyle type="line" width="1.0" color="#000000"/>
<y:Arrows source="none" target="standard"/>
<y:EdgeLabel alignment="center" backgroundColor="#FFFFFF" configuration="AutoFlippingLabel" distance="2.0" fontFamily="Dialog" fontSize="12" fontStyle="plain" hasLineColor="false" height="17.96875" horizontalTextPosition="center" iconTextGap="4" modelName="centered" preferredPlacement="anywhere" ratio="0.5" textColor="#000000" verticalTextPosition="bottom" visible="true"><foaf:givenName></y:EdgeLabel>
<y:BendStyle smoothed="false"/>
</y:PolyLineEdge>
</data></edge>
<node id="n3"><data key="d0">
<y:ShapeNode>
<y:Geometry x="170.5" y="-15.0" width="110.0" height="30.0"/>
<y:Fill color="#CCFFCC" transparent="false"/>
<y:BorderStyle type="line" width="1.0" color="#000000"/>
<y:NodeLabel>"Skywalker"</y:NodeLabel>
<y:Shape type="ellipse"/>
</y:ShapeNode>
</data></node>
<edge source="n0" target="n3"><data key="d1">
<y:PolyLineEdge>
<y:Path sx="0.0" sy="-15.0" tx="29.5" ty="0.0">
<y:Point x="425.0" y="0.0"/>
</y:Path>
<y:LineStyle type="line" width="1.0" color="#000000"/>
<y:Arrows source="none" target="standard"/>
<y:EdgeLabel alignment="center" backgroundColor="#FFFFFF" configuration="AutoFlippingLabel" distance="2.0" fontFamily="Dialog" fontSize="12" fontStyle="plain" hasLineColor="false" height="17.96875" horizontalTextPosition="center" iconTextGap="4" modelName="centered" preferredPlacement="anywhere" ratio="0.5" textColor="#000000" verticalTextPosition="bottom" visible="true"><foaf:familyName></y:EdgeLabel>
<y:BendStyle smoothed="false"/>
</y:PolyLineEdge>
</data></edge>
<node id="n4"><data key="d0">
<y:ShapeNode>
<y:Geometry x="170.5" y="-15.0" width="190.0" height="30.0"/>
<y:Fill color="#CCFFCC" transparent="false"/>
<y:BorderStyle type="line" width="1.0" color="#000000"/>
<y:NodeLabel>"23"^^<xsd:integer></y:NodeLabel>
<y:Shape type="ellipse"/>
</y:ShapeNode>
</data></node>
<edge source="n0" target="n4"><data key="d1">
<y:PolyLineEdge>
<y:Path sx="0.0" sy="-15.0" tx="29.5" ty="0.0">
<y:Point x="425.0" y="0.0"/>
</y:Path>
<y:LineStyle type="line" width="1.0" color="#000000"/>
<y:Arrows source="none" target="standard"/>
<y:EdgeLabel alignment="center" backgroundColor="#FFFFFF" configuration="AutoFlippingLabel" distance="2.0" fontFamily="Dialog" fontSize="12" fontStyle="plain" hasLineColor="false" height="17.96875" horizontalTextPosition="center" iconTextGap="4" modelName="centered" preferredPlacement="anywhere" ratio="0.5" textColor="#000000" verticalTextPosition="bottom" visible="true"><foaf:age></y:EdgeLabel>
<y:BendStyle smoothed="false"/>
</y:PolyLineEdge>
</data></edge>
<node id="n5"><data key="d0">
<y:ShapeNode>
<y:Geometry x="170.5" y="-15.0" width="220.0" height="30.0"/>
<y:Fill color="#E1E1E1" transparent="false"/>
<y:BorderStyle type="line" width="1.0" color="#000000"/>
<y:NodeLabel>_:n7235299428451853102</y:NodeLabel>
<y:Shape type="ellipse"/>
</y:ShapeNode>
</data></node>
<edge source="n5" target="n1"><data key="d1">
<y:PolyLineEdge>
<y:Path sx="0.0" sy="-15.0" tx="29.5" ty="0.0">
<y:Point x="425.0" y="0.0"/>
</y:Path>
<y:LineStyle type="line" width="1.0" color="#000000"/>
<y:Arrows source="none" target="standard"/>
<y:EdgeLabel alignment="center" backgroundColor="#FFFFFF" configuration="AutoFlippingLabel" distance="2.0" fontFamily="Dialog" fontSize="12" fontStyle="plain" hasLineColor="false" height="17.96875" horizontalTextPosition="center" iconTextGap="4" modelName="centered" preferredPlacement="anywhere" ratio="0.5" textColor="#000000" verticalTextPosition="bottom" visible="true"><rdf:type></y:EdgeLabel>
<y:BendStyle smoothed="false"/>
</y:PolyLineEdge>
</data></edge>
<edge source="n5" target="n0"><data key="d1">
<y:PolyLineEdge>
<y:Path sx="0.0" sy="-15.0" tx="29.5" ty="0.0">
<y:Point x="425.0" y="0.0"/>
</y:Path>
<y:LineStyle type="line" width="1.0" color="#000000"/>
<y:Arrows source="none" target="standard"/>
<y:EdgeLabel alignment="center" backgroundColor="#FFFFFF" configuration="AutoFlippingLabel" distance="2.0" fontFamily="Dialog" fontSize="12" fontStyle="plain" hasLineColor="false" height="17.96875" horizontalTextPosition="center" iconTextGap="4" modelName="centered" preferredPlacement="anywhere" ratio="0.5" textColor="#000000" verticalTextPosition="bottom" visible="true"><foaf:knows></y:EdgeLabel>
<y:BendStyle smoothed="false"/>
</y:PolyLineEdge>
</data></edge>
<node id="n6"><data key="d0">
<y:ShapeNode>
<y:Geometry x="170.5" y="-15.0" width="60.0" height="30.0"/>
<y:Fill color="#CCFFCC" transparent="false"/>
<y:BorderStyle type="line" width="1.0" color="#000000"/>
<y:NodeLabel>"Leia"</y:NodeLabel>
<y:Shape type="ellipse"/>
</y:ShapeNode>
</data></node>
<edge source="n5" target="n6"><data key="d1">
<y:PolyLineEdge>
<y:Path sx="0.0" sy="-15.0" tx="29.5" ty="0.0">
<y:Point x="425.0" y="0.0"/>
</y:Path>
<y:LineStyle type="line" width="1.0" color="#000000"/>
<y:Arrows source="none" target="standard"/>
<y:EdgeLabel alignment="center" backgroundColor="#FFFFFF" configuration="AutoFlippingLabel" distance="2.0" fontFamily="Dialog" fontSize="12" fontStyle="plain" hasLineColor="false" height="17.96875" horizontalTextPosition="center" iconTextGap="4" modelName="centered" preferredPlacement="anywhere" ratio="0.5" textColor="#000000" verticalTextPosition="bottom" visible="true"><foaf:givenName></y:EdgeLabel>
<y:BendStyle smoothed="false"/>
</y:PolyLineEdge>
</data></edge>
<node id="n7"><data key="d0">
<y:ShapeNode>
<y:Geometry x="170.5" y="-15.0" width="80.0" height="30.0"/>
<y:Fill color="#CCFFCC" transparent="false"/>
<y:BorderStyle type="line" width="1.0" color="#000000"/>
<y:NodeLabel>"Organa"</y:NodeLabel>
<y:Shape type="ellipse"/>
</y:ShapeNode>
</data></node>
<edge source="n5" target="n7"><data key="d1">
<y:PolyLineEdge>
<y:Path sx="0.0" sy="-15.0" tx="29.5" ty="0.0">
<y:Point x="425.0" y="0.0"/>
</y:Path>
<y:LineStyle type="line" width="1.0" color="#000000"/>
<y:Arrows source="none" target="standard"/>
<y:EdgeLabel alignment="center" backgroundColor="#FFFFFF" configuration="AutoFlippingLabel" distance="2.0" fontFamily="Dialog" fontSize="12" fontStyle="plain" hasLineColor="false" height="17.96875" horizontalTextPosition="center" iconTextGap="4" modelName="centered" preferredPlacement="anywhere" ratio="0.5" textColor="#000000" verticalTextPosition="bottom" visible="true"><foaf:familyName></y:EdgeLabel>
<y:BendStyle smoothed="false"/>
</y:PolyLineEdge>
</data></edge>
</graph>
</graphml>
[
{"subject":"_:n7510155278594416037","predicate":"\u003crdf:type\u003e","object":"\u003cfoaf:Person\u003e","label":"people"},
{"subject":"_:n7510155278594416037","predicate":"\u003cfoaf:givenName\u003e","object":"Luke","label":"people"},
{"subject":"_:n7510155278594416037","predicate":"\u003cfoaf:familyName\u003e","object":"Skywalker","label":"people"},
{"subject":"_:n7510155278594416037","predicate":"\u003cfoaf:age\u003e","object":"\"23\"^^\u003cxsd:integer\u003e","label":"people"},
{"subject":"_:n7235299428451853102","predicate":"\u003crdf:type\u003e","object":"\u003cfoaf:Person\u003e","label":"people"},
{"subject":"_:n7235299428451853102","predicate":"\u003cfoaf:knows\u003e","object":"_:n7510155278594416037","label":"people"},
{"subject":"_:n7235299428451853102","predicate":"\u003cfoaf:givenName\u003e","object":"Leia","label":"people"},
{"subject":"_:n7235299428451853102","predicate":"\u003cfoaf:familyName\u003e","object":"Organa","label":"people"}
]
_:n7510155278594416037 <rdf:type> <foaf:Person> "people" .
_:n7510155278594416037 <foaf:givenName> "Luke" "people" .
_:n7510155278594416037 <foaf:familyName> "Skywalker" "people" .
_:n7510155278594416037 <foaf:age> "23"^^<xsd:integer> "people" .
_:n7235299428451853102 <rdf:type> <foaf:Person> "people" .
_:n7235299428451853102 <foaf:knows> _:n7510155278594416037 "people" .
_:n7235299428451853102 <foaf:givenName> "Leia" "people" .
_:n7235299428451853102 <foaf:familyName> "Organa" "people" .
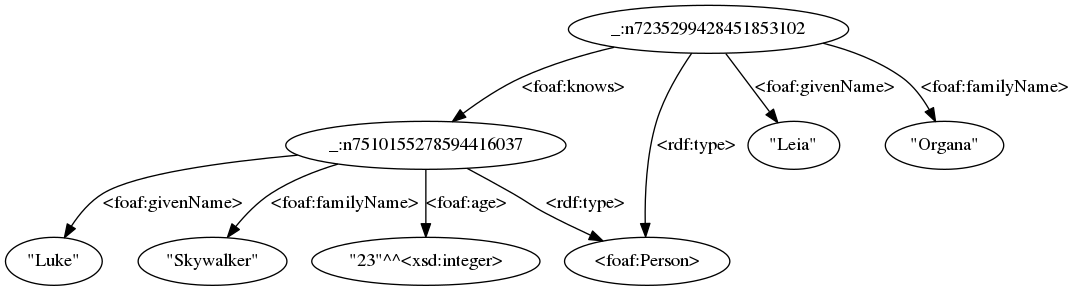
Rendered Graphviz
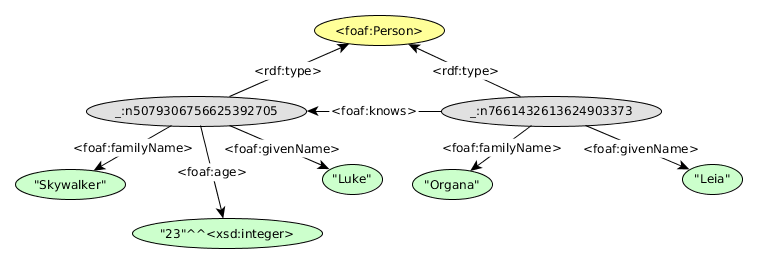